Pippy
see more templates or propose new |
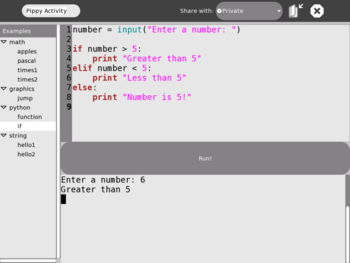
Contents
Description & Goals
Summary
Teaches Python programming by providing access to Python code samples and a fully interactive Python interpreter.
The user can type and execute simple Python expressions. For example, it would be possible for a user to write Python statements to calculate expressions, play sounds, or make simple text animation.
The initial build ships with about twenty short Python examples covering various aspects of the language.
Goals
To introduce children to computer programming
Collaboration
?
Examples
Please add examples here, or modify the existing ones!
Math
Apples
- Author: Madeleine Ball
- About: Adding and dividing
- Shows: Print Statements and Basic Math
print "Let's do math!" print "On Monday I picked 22 apples. On Tuesday I picked 12." print "Now I have: ", 22 + 12 print "My brother says he picked twice as many apples last week." print "This means he picked: ", (22 + 12) * 2 print "I have 3 friends I would like to give apples." print "One third of my apples is about: ", (22 + 12) / 3 print "Or, more exactly: ", (22.0 + 12.0) / 3.0
Pascal's triangle
- Author: Madeleine Ball
- About: Character graphic of Pascal's triangle
- Shows: loops, vectors
# Pascal's triangle lines = 8 vector = [1] for i in range(1,lines+1): vector.insert(0,0) vector.append(0) for i in range(0,lines): newvector = vector[:] for j in range(0,len(vector)-1): if (newvector[j] == 0): print " ", else: print "%2d" % newvector[j], newvector[j] = vector[j-1] + vector[j+1] print vector = newvector[:]
Sierpinski triangle
- Author: Madeleine Ball
- About: Character graphics of a Sierpinski triangle
- Shows: Modifying Pascal's triangle program, loops, vectors
size = 5 modulus = 2 lines = modulus**size vector = [1] for i in range(1,lines+1): vector.insert(0,0) vector.append(0) for i in range(0,lines): newvector = vector[:] for j in range(0,len(vector)-1): if (newvector[j] == 0): print " ", else: remainder = newvector[j] % modulus if (remainder == 0): print "O", else: print ".", newvector[j] = vector[j-1] + vector[j+1] print vector = newvector[:]
Times1
- Author: Chris Ball
- About: The 4 times table
- Shows: Loops, the range statement
for i in range(1,13): print i, "x 4 =", (i*4)
Times2
- Author: Chris Ball
- About: Print any times table
- Shows: Loops, range, and input
number = input("Which times table? ") for i in range(1,13): print i, "x", number, "=", i*number
Fibonacci Series
- Author : Rafael Ortiz
- About: The Fibonacci Number Series
- Shows: Using tuple assignments. While loop.
a, b = 0, 1 while b < 1001: print b, a, b = b, a+b
Pythagoras
- Author : Rafael Ortiz
- About: Uses Pythagorean Theorem to compute longest edge of a triangle
- Shows: Import statement, sqrt (square root) function.
import math from math import sqrt print "This is the Pythagoras Theorem" a=float(raw_input("Type a =")) b=float(raw_input("Type b =")) c=sqrt((a*a)+(b*b)) print "c =",c
Factorize
- Author: Reinier Heeres
- About: Factoring Numbers with trial divisions
- Shows: Appending to arrays, import, sys.stdout
import math import sys orignum = input("Enter a number to factorize ") factors = [] num = orignum i = 2 while i <= math.sqrt(num): if num % i == 0: factors.append(i) num /= i i = 2 elif i == 2: i += 1 else: i += 2 factors.append(num) if len(factors) == 1: print "%d is prime" % orignum else: sys.stdout.write("%d is %d" % (orignum, factors[0])) for fac in factors[1:]: sys.stdout.write(" * %d" % fac) print
Zeros of a second degree polynomial
- Author: Pilar Saenz
- About: Zeros of a second grade polynomial, e.g., 3x^2+6x+3.
- Shows: Converting strings to float, import, sqrt (square root)
import math from math import sqrt print "These are the zeros of a second grade polynomial" a=float(raw_input("Type a =")) b=float(raw_input("Type b =")) c=float(raw_input("Type c =")) aux=b*b-4*a*c; if aux>0: x1=(-b+sqrt(aux))/(2*a) x2=(-b-sqrt(aux))/(2*a) print "x1= " , x1 ,", x2=" ,x2 elif aux==0: print "x= " , -b/(2*a) else: x1=(-b+sqrt(-aux)*1j)/(2*a) x2=(-b+sqrt(-aux)*1j)/(2*a) print "x1= " , x1 , ", x2" , x2
Factorial of a number
- Author: Pilar Saenz
- About: Prints a factorial
- Shows: Defining and calling a function. Casting to int.
def factorial(a): fac=a for i in range(1,a): fac=fac*i print a,"!=",fac a=int(raw_input("Type a=")) factorial(a)
Greatest common divisor
- Author: Pilar Saenz
- About: The greatest common divisor of two numbers
- Shows: The % (modulo) operator
n= input("Enter a number ") m= input("Enter another number ") r=n%m if r!=0: while (r!=0): n=m m=r r=n%m print "The greatest common divisor is ", m
Python
Function
Author: Chris Ball
def square(x): print x * x square(3) square(4)
If
Author: Chris Ball
number = input("Enter a number: ") if number > 5: print "Greater than 5" elif number < 5: print "Less than 5" else: print "Number is 5!"
Recursion
Author: Mel Chua
# Note this assumes you understand functions and if-else. def countbackwards(number): print "I have the number", number if number > 0: print "Calling countbackwards again!" countbackwards(number-1) else: print "I am done counting" number = input("Enter a number: ") countbackwards(number):
While
Author Pilar Saenz
n=input("enter a number") while n>0: print n, " ", n=n-1 print "Surprise!\n"
Title Case Capitalisation
Author: Alan Davies
# This is an example of a list comprehension oldtitle = "this TITLE iS NOW coRRecTly CAPItalised" oldwords = oldtitle.split() newwords = [word[0].upper() + word[1:].lower() for word in oldwords] newtitle = " ".join(newwords) print "Before:", oldtitle print "After:", newtitle
Names Drawn From a Hat
Author: Alan Davies
# Simple and possibly useful program for # drawing names in a random order from a hat import random names = [] name = raw_input("Enter the first name to go in the hat:") while name != "": names.append(name) name = raw_input("Enter another name, leave blank if you have finished:") random.shuffle(names) print "The random order from the hat is:" for x in range(len(names)): print x+1, names[x]
String
Hello1
Author: Chris Ball
print "Hello everyone!"
Hello2
Author: Chris Ball
name = raw_input("Type your name here: ") print "Hello " + name + "!"
Thanks
Author: Walter Bender
Comment: Please add names as appropriate
import random from random import choice table = { 'Hardware & Mechanicals': 'John Watlington, Mark Foster, Mary Lou Jepsen, Yves Behar, Bret Recor, Mitch Pergola, Martin Schnitzer, Kenneth Jewell, Kevin Young, Jacques Gagne, Nicholas Negroponte, Frank Lee, Victor Chau, Albert Hsu, HT Chen, Vance Ke, Ben Chuang, Johnson Huang, Sam Chang, Alex Chu, Roger Huang, and the rest of the Quanta team, the Marvell team, the AMD team, the ChiMei team..', 'Firmware': 'Ron Minnich, Richard Smith, Mitch Bradley, Tom Sylla, Lilian Walter, Bruce Wang..', 'Kernel & Drivers': 'Jaya Kumar, Jon Corbet, Reynaldo Verdejo, Pierre Ossman, Dave Woodhouse, Matthew Garrett, Chris Ball, Andy Tanenbaum, Linus Torvalds, Dave Jones, Andres Salomon, Marcelo Tosatti..', 'Graphics systems': 'Jordan Crouse, Daniel Stone, Zephaniah Hull, Bernardo Innocenti, Behdad Esfahbod, Jim Gettys, Adam Jackson, Behdad Esfahbod..', 'Programming': 'Guido Van Rossum, Johan Dahlin, Brian Silverman, Alan Kay, Kim Rose, Bert Freudenberg, Yoshiki Ohshima, Takashi Yamamiya, Scott Wallace, Ted Kaehler, Stephane Ducasse, Hilaire Fernandes..', 'Sugar': 'Marco Pesenti Gritti, Dan Williams, Chris Blizzard, John Palmieri, Lisa Strausfeld, Christian Marc Schmidt, Takaaki Okada, Eben Eliason, Walter Bender, Tomeu Vizoso, Simon Schampijer, Reinier Heeres, Ben Saller, Miguel Alvarez..', 'Activities': 'Erik Blankinship, Bakhtiar Mikhak, Manusheel Gupta, J.M. Maurer (uwog) and the Abiword team, the Mozilla team, Jean Piche, Barry Vercoe, Richard Boulanger, Greg Thompson, Arjun Sarwal, Cody Lodrige, Shannon Sullivan, Idit Harel, and the MaMaMedia team, John Huang, Bruno Coudoin, Eduardo Silva, H&?kon Wium Lie, Don Hopkins, Muriel de Souza Godoi, Benjamin M. Schwartz..', 'Network': 'Michael Bletsas, James Cameron, Javier Cardona, Ronak Chokshi, Polychronis Ypodimatopoulos, Simon McVittie, Dafydd Harries, Sjoerd Simons, Morgan Collett, Guillaume Desmottes, Robert McQueen..', 'Security': 'Ivan Krstic, Michael Stone, C. Scott Ananian, Noah Kantrowitz, Herbert Poetzl, Marcus Leech..', 'Content': 'SJ Klein, Mako Hill, Xavier Alvarez, Alfonso de la Guarda, Sayamindu Dasgupta, Mallory Chua, Lauren Klein, Zdenek Broz, Felicity Tepper, Andy Sisson, Christine Madsen, Matthew Steven Carlos, Justin Thorp, Ian Bicking, Christopher Fabian, Wayne Mackintosh, the OurStories team, Will Wright, Chuck Normann..', 'Testing': 'Kim Quirk, Alex Latham, Giannis Galanis, Ricardo Carrano, Zach Cerza, John Fuhrer..', 'Country Support': 'Carla Gomez Monroy, David Cavallo, Matt Keller, Khaled Hassounah, Antonio Battro, Audrey Choi, Habib Kahn, Arnan (Roger) Sipitakiat', 'Administrative Support': 'Nia Lewis, Felice Gardner, Lindsay Petrillose, Jill Clarke, Julia Reynolds, Tracy Price, David Robertson, Danny Clark', 'Finance & Legal': 'Eben Moglen, Bruce Parker, William Kolb, John Sare, Sandra Lee, Richard Bernstein, Jaclyn Tsai, Jaime Cheng, Robert Fadel, Charles Kane (Grasshopper), Kathy Paur, Andriani Ferti', 'PR and Media': 'Larry Weber, Jackie Lustig, Jodi Petrie, George Snell, Kyle Austin, Hilary Meserole, Erick A. Betancourt, Michael Borosky, Sylvain Lefebvre, Martin Le Sauteur', 'Directors & Advisors': 'Howard Anderson, Rebecca Allen, Ayo Kusamotu, Jose Maria Aznar, V. Michael Bove, Jr., Rodrigo Mesquita, Seymour Papert, Ted Selker, Ethan Beard (Google); John Roese (Nortel); Dandy Hsu (Quanta); Marcelo Claure (Brightstar); Gary Dillabough (eBay); Gustavo Arenas (AMD); Mike Evans (Red Hat); Ed Horowitz (SES Astra); Jeremy Philips (NewsCorp); Scott Soong (Chi Lin); Sehat Sutardja (Marvell); Joe Jacobson (MIT Media Lab); Steve Kaufman (Riverside); and Tom Meredith (MFI)', 'Pippy': 'Chris Ball' } print "OLPC would like to take this opportunity to acknowledge the community of people and projects that have made the XO laptop possible." subsystem = random.choice(table.keys()); print subsystem, '\t',table[subsystem]
Graphics
Jump
Author: C. Scott Ananian
# both of these functions should be in the 'basic' package or some such def clear_scr(): print '\x1B[H\x1B[J' # clear screen, the hard way. def wait(): import time time.sleep(0.1) # jumping man! # was having to escape the backslash which was rather unfortunate, # now using python's r" strings which were meant for regex's # i didn't have to do that in C64 BASIC for i in xrange(0,50): clear_scr() print r"\o/" print r"_|_" print r" " wait() clear_scr() print r"_o_" print r" | " print r"/ \ " wait() clear_scr() print r" o " print r"/|\ " print r"| |" wait() clear_scr() print r"_o_" print r" | " print r"/ \ " wait()
Mandelbrot Set
Author: Alan Davies
# Text-based Mandelbrot set generator # Play with the values of 'centre' and 'realsize' # to explore the set. centre, realsize, maxiter = -.7+0j, 2.8, 50 width, height, aspect = 60, 30, 1.9 charmap = "abcdefghijklmnopqrstuvwxyz" for y in range(height): output = "" for x in range(width): real = (float(x)/width-.5)*realsize imag = (float(y)/height-.5)*aspect*realsize*height/width z = c = complex(real, imag) + centre iterations = 0 while abs(z) < 2 and iterations < maxiter: z = z**2 + c iterations += 1 if iterations == maxiter: output += " " else: output += charmap[iterations%len(charmap)] print output
Games
Guess a number
Author: Pilar Saenz
import random from random import randrange R = randrange(1,100) print "Guess a number between 1 and 100!!!" N = input("Enter a number: ") i=1 while (N!=R): if N>R : print "Too big... try again" else : print "Too small.. try again" N = input("Enter a number: ") i=i+1 print "You got it in ", i, "tries"
Robots
Author: Alan Davies
This is a playable implementation of Robots (also known as Daleks). I tried to keep the code clear and well commented, even at the expense of space. I also made sure that the lines don't wrap in Pippy, as that looks quite ugly.
I'm not sure if this is considered too large for the samples to be included with Pippy- a simpler implementation could be trimmed down considerably. I figured it might be nice to have at least one complete implementation for kids and adults to play with.
from random import randint import curses stdscr = curses.initscr() curses.noecho() xmax, ymax, alive = 60, 10, True commands = {"q":(-1,-1), "w":(0,-1), "e":(1,-1), "a":(-1,0), "s":(0,0), "d":(1,0), "z":(-1,1), "x":(0,1), "c":(1,1), " ":(0,0), "t":(0,0)} def message(text, yoffset=0, wait=True): stdscr.addstr(ymax/2+yoffset, xmax/2-len(text)/2, text) stdscr.refresh() if wait: stdscr.getch() message("Welcome to Robots!", -3, False) message(" QWE Screwdriver: S ", -1, False) message("Movement: A D Teleport: T ", 0, False) message(" ZXC Do nothing: Spacebar", 1, False) message("Press any key...", 3) while True: level = 1 alive = True while alive: # Initialise powers, hero position, and enemy lists teleport = screwdriver = True hero = (xmax/2, ymax/2) scrap = [(randint(0, xmax), randint(0, ymax)) for dummy in range(level/3+3)] robots = [(randint(0, xmax), randint(0, ymax)) for dummy in range(level*4-3)] scrap = [s for s in scrap if s != hero] robots = [r for r in robots if r != hero] while True: # move crashed robots to scrap list scrap += [r for r in robots if robots.count(r) >= 2] robots = [r for r in robots if scrap.count(r) == 0] # draw the screen stdscr.clear() stdscr.addstr(hero[1], hero[0], "@") for robot in robots: stdscr.addstr(robot[1], robot[0], "$") for scr in scrap: stdscr.addstr(scr[1], scr[0], "#") if screwdriver: stdscr.addstr(ymax-1, xmax+1, "S") stdscr.addstr(ymax, xmax+1,"T" if teleport else "") stdscr.refresh() # test for win or loss if len(robots) == 0: break elif hero in robots or hero in scrap: stdscr.addstr(hero[1], hero[0], "X") message("You lost! Press any key...") alive = False break # get a valid keypress key = "" while key not in commands: key = chr(stdscr.getch()).lower() # teleport - move to a random location if teleport and key == "t": teleport = False hero = (randint(0, xmax), randint(0, ymax )) # sonic screwdriver - scraps nearby robots if screwdriver and key == "s": screwdriver = False scrap += [robot for robot in robots if abs(robot[0] - hero[0]) <= 1 and abs(robot[1] - hero[1]) <= 1] robots = [r for r in robots if scrap.count(r) == 0] # update hero and robot positions hero = (hero[0] + commands[key][0], hero[1] + commands[key][1]) def sign(x): return (x / abs(x)) if x else 0 def follow(fr, to): return (fr[0] + sign(to[0]-fr[0]), fr[1] + sign(to[1]-fr[1])) robots = [follow(robot, hero) for robot in robots] # move to next level if alive: level += 1 message(" Level %d! Press any key... " % level)
compute pi!
Author: Travis Hall
Simple pi computation demo, does 1000 loops... to do more loops change the breakpoint varible to a higher number. Has a 11 decimal limit, can anyone increase it??? (I think it may be a limit of Pippy...)
a,b = 1.0,3.0 loop = 1 breakpoint = 1000 pi = 0.0 while loop < breakpoint: pi = pi + (4.0/a) - (4.0/b); a = a + 4; b = b + 4; print pi; loop = loop + 1;
Beginning Programming
C. Scott Ananian created a small library of programming examples, based on the BASIC examples in the Commodore 64 manual. It can be found at http://dev.laptop.org/git?p=users/cscott/pippy-examples;a=tree