Display
The display is one of the innovative features of the XO laptop. It can be used in darkness, and also in direct sunlight. Because learning takes place in both.
Introduction
The interesting features of the display system include:
- high resolution
- sunlight readable black & white mode
- innovative color pixel layout optimized for both portrait and landscape modes
- low power operation
These features introduce some special considerations when generating graphics for the display, introduced in designing for the display. A later section delves into more implementation details.
Designing for the display
Quick Summary
The display has a resolution of 1200 x 900. It's size is 7.5" diagonal, or 6 x 4 inches (152.4 x 114.3 mm), which gives a dot pitch of 200 dpi. It supports a color resolution of approximately 6 bits per color (262k colors).
Use normal font point sizes.
UI elements designed for 100 dpi should be enlarged by about 1/3, or they will look too small.
Under different lighting conditions, the display may appear to be color, pale color, or monochrome. So check the appearance of your UI in monochrome. Use high-contrast UI elements. Pick colors with different luminances.
How to switch to reflective mode: press the "lower brightness" button repeatedly until the backlight is turned off.
How to switch to color mode: press the "increase brightness" button to turn the backlight on.
Elaboration
Use normal font point sizes. That's points, not pixels, of course. The fonts will appear larger than normal (because the child is closer than usual). But compared with adults, children use larger fonts. So a 12 pt font looking 14 pt is fine.
Ignore talk of "mono and color modes", and of screen resolutions other than 1200x900. We named things poorly, and immense confusion has resulted. We called two very different things mono/color. Which combined with the unusual screen hardware, has generated immense confusion,and consequent misinformation. The hardware section below discusses it, but most activities just don't care.
UI design and physical geometry
Think about how large your UI elements will actually be in physical geometry, and design accordingly. Normal viewing distance might be 40-50 cm. Compared to 40 to 60 or 70 cm for adults. Pixel size is 0.127 mm, or about 1 arc minute. Elements designed for 100 dpi should be enlarged 20-50%, or they will look smaller. 1/3 is a nice number. The web browser scales web pages by ~40%. (what is the precise browser number?)
You only really need to worry about the color artifacts if you're trying to draw elements smaller than 3 pixels wide or tall. But that's probably a bad idea anyway, since that's going to be tiny (0.01 in, 0.24 mm).
Color selection tools
LUV and Lab color pickers and palettes may be helpful for choosing colors. The XO can be colorful. You just have one extra consideration, luminance, to manage. A Munsell-based palette is another option.
The following code can be useful to separate gray-level and color decisions.
import colorsys def gray_rgb(gray,r,g,b): "Given an rgb and a gray level, return a similar rgb with gray-like intensity". h,l,s = colorsys.rgb_to_hls(r,g,b) return colorsys.hls_to_rgb(h,gray,s)
There is another implementation: [1]. It returns the wrong thing for a different set of inputs, but is not noticeably better. And it's much more complex.
Use
- You can always at least see grayscale, even in direct sunlight.
- You get color from the backlight. Though as sunlight gets brighter, the colors wash out and it again looks like grayscale.
- The backlight uses power. So you can turn it down, and off, to make the battery last longer.
- Turning off the backlight also tells the screen to not worry about color, so it can give you a slightly higher resolution. Which can make hours of reading more comfortable.
Understanding the display system
The display has two main parts, the screen, and the DCON screen driver chip.
Screen
The screen is unusual. It can be used in darkness and in direct sunlight. There are several ways to describe it.
Our screen, described as two screens sharing an LCD
It is important to remember in the following discussion that the system frame buffer stores a full 16 bits (XO-1)/24 bits (XO-1.5) for each pixel on the display.
One way to think of the screen is as the combination of two separate screens, which share only an LCD glass. One screen is a normal backlit color screen. The other is a normal monochromatic reflective screen.
The LCD is a 1200x900 grid of square, 0.127 mm (200 dpi) pixels which each have 64 levels of gray (6 bits). As usual, when a pixel is off, it's transparent. And when it is fully on, it is opaque.
The backlit "color screen" has a backlight that shines through a fixed color filter onto the 1200x900 grid. The filter lights each pixel with just one permanent color: red, green, or blue. The intensity of the color is determined by the LCD gray level which is calculated by taking into account neighboring pixels.<ref>DCON filter kernel</ref><ref>DCON Specification</ref>
The reflective "monochrome screen" has a reflector behind the LCD grid. So room light comes in (through the LCD), bounces off the reflector, and goes back out, through the LCD. So there are 1200x900 pixels, which depend on ambient outside light to be seen.
The light the user sees comes from both of these. Some from the reflective "monochrome screen", and some from the backlit "color screen". How much comes from each depends on the backlight brightness setting and the ambient brightness of the room/outdoors.
In a completely dark room, you see only the backlit "color screen". In direct sunlight, you only see the reflective "monochrome screen". In between, you see a variable mix of both.
If you reflect the luminance in all pixels, and the primary colors are sub-sampled by three, what's the resolution of the combined screen? In sunlight, or in a normal room with the backlight turned off, it's 1200x900. Under normal conditions, with the backlight on, perception tests put it at something like XGA (which is 1024x768). Why use perception tests? Because the whole emphasis of the unusual screen design is to mesh well with how human perception works. So to get a useful measurement, you need to include an eyeball.
Our screen, described by its parts
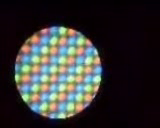
The screen is composed of several layers. Starting at the back, there is a white LED backlight, a 1200x900 grid of color filters, a semi-reflective layer, and a 1200x900 LCD.
The brightness of the backlight can be adjusted. It has 16 settings, including off.
The semi-reflective layer both reflects room light and lets the backlight's color-filtered light shine through. How much you see of each source depends on the relative strength of the two light sources.
- In direct sun, you see only reflected, monochromatic light. The backlight setting doesn't matter.
- In a completely dark room, there is no reflected light. So you only see the color-filtered backlight, and if you turn it off, you see nothing.
- In between, you see some mix. You see more color-filtered backlight if you turn down the room lighting, or you raise the backlight setting. You see less color-filtered backlight when the room gets brighter, or you lower the backlight setting.
All color is created by the backlight and filters.
There are 1200x900 pixels. Each one has a single colored filter behind it. So each pixel shows one primary color of either R, G, or B. Only one. It relies on its neighbors to provide the other primary colors. So each pixel has:
- a fixed hue (R, G, or B),
- a luminance which can be set (6 bit)
- and a chrominance which depends on the relative strength of the room light and backlight.
This Munsell page has a nice diagram.
Consider a single one of those 1200x900 pixels. A red one. If its value is 0, black, then lighting doesn't matter. If its value is 255 (or whatever, fully transparent), then in bright sunlight you see only white, and in a dark room you see fully saturated red. If its value is in between, in bright sunlight you see a gray, and in a dark room you see a grayed (i.e., desaturated) red.
The theory
The display employs something the video encoding experts have done for some time: the human visual system sees higher resolution in luminance (B&W) than chrominance (color): for example MPEG movies have luminance resolution that is 4X greater than the chrominance resolution.<ref name="Livingstone">Margaret Livingstone; David Hubel (2002). Vision and Art: The Biology of Seeing. Harry N. Abrams. pp. 208. ISBN 0810904063. http://www.worldcat.org/wcpa/oclc/47745847.</ref> The OLPC XO-1 display, similarly, offers higher resolution luminance information than chrominance. A key thing to understand is that the blend of information, and thus the perceived resolution of the display, varies as the ambient light level of the room changes. Each pixel has both a reflective part that is B&W, and a transmissive part that is one color: red or green or blue. If one red, one green and one blue pixel merely combined to make a single full-color pixel, then the resolution would be 1200/sqrt(3) x 900/sqrt(3) or 693x520.
The perceived resolution when viewed in a totally dark room, however, is approximately 984×738 or about 164 dpi when measured using standard methods of determining display resolution. In a dark room the effect is akin to sub-pixel rendering and we measure an improvement in resolution of ~100% due to this effect. These measurements were done in a number of ways and were written up for publication by the Society for Information Display 2008 Annual Meeting (some were straight fresnel patterns, others were perceptual image detail tests).<ref>Mary Lou Jepsen (May 27, 2008). "Template:Citation error". Archived from the original. Template:Citation error. https://web.archive.org/web/20110715085536/http://www.pixelqi.com/blog1/2008/05/27/higher-resolution-than-we-thought-the-xo-laptop-screen/. Retrieved on 2009-10-27.</ref><ref name="SID08">Klompenhouwer, Michiel; Erno H.A. Langendijk (2008-05-27). Comparing the Effective Resolution of Various RGB Subpixel Layouts. Los Angeles, California: Society for Information Display Annual Meeting. doi: . SID08. http://www.sid.org/conf/sid2008/program/symposium.html. Retrieved on 2008-06-16.</ref>
With room lights on, the display's reflective mode now shows luminance (B&W) information at 200 dpi as well as chrominance (color) information from the transmissive mode. The combination increases the effective resolution to about XGA or 1024x768 or about 176 dpi when using test patterns to ascertain the display resolution.
Finally, the laptop can be brought outside into bright sunlight and the screen is still viewable - now the color is barely visible (if the backlight is left on), but on the screen the 1200x900 or 200 dpi resolution is seen crisply and clearly.
Other notes
The top left corner pixel is red. So the first row is RGBRG... and the second row is GBRGB... and the 3rd BRGBR... .
RGBRGB...
GBRGBR...
BRGBRG...
.
.
The luminance of the display, in a completely dark environment, is typically 85 cd/m^2 (which is very bright in a dark environment), with a contrast ratio of 85:1. In a dimly lit room (140 lux), the luminance is 100 cd/m^2. In a brightly lit room (350 lux), the display's luminance is 175 cd/m^2. In sunlight (2000 lux), the display's luminance is 590 cd/m^2. The beauty of a transflective display is that as the ambient illumination increases, the luminance of the display increases.
The frame buffer is always 1200x900, 200 dpi. Always. What that 200 dpi looks like varies a great deal depending on lighting. Lit externally, you see the grayscale pixels on a 1/200 inch grid. Lit internally (by the backlight), the display gains color. Depending on the ratio of external to internal lighting, the pixels vary from pure gray (black-to-white), to tinted, to pure color (black-to-... red, green or blue, depending on the pixel).
DCON screen driver chip
The DCON operates in one of two modes:
- monochrome, used when the backlight is off
- color swizzled anti-aliased, used when the backlight is on
Additional modes (such as color swizzled but not anti-aliases, and pass-through) are supported but not used in normal operation.
Control from Linux
Using Sugar, or olpc-hardware-manager, to set /sys/devices/platform/dcon/output to 0 (color), gives "DCON color swizzled antialised". Setting it to 1 (mono), gives you DCON monochrome mode. You cannot currently get "DCON color swizzled not antialised".
For each pixel, the DCON always gets 19 bits input (6-7-6) from the frame buffer, and outputs a 6 bit pixel transparency for that pixel color component.
In DCON monochrome mode, the pixel's 6 bits come from a normal luminance calculation: y = 0.312*r + 0.563*g + 0.125*b.
Swizzling means that you select only one of the r,g,b channels. The color of the filter which the pixel is in front of. The other two are ignored (unless antialiasing is turned on).
In antialiasing, in addition to considering one's own channel value, you also consider the values of that channel on adjacent pixels. Anti-aliasing is enabled by default in XO system software.
ICC Profile
An ICC output profile of the display is available: File:OLPC icc.zip
See also
- Hardware specification#Display
- The source code for the Geode X Window System display driver is at http://cgit.freedesktop.org/xorg/driver/xf86-video-geode/
- DCON and its File:DCON Specification, V0.8.odt
- Dealing with Dual-Mode Display
- Munsell
- http://lists.laptop.org/pipermail/devel/2007-January/003817.html
- #1017 has some discussion of the color pipeline
- http://croquetweak.blogspot.com/2007/03/interactive-olpc-xo-display-simulation.html
- Talk:Hardware_specification#Display_characterization_.28as_we_outsiders_understand_it.29 Warning - parts of this are misleading/mistaken.
- http://dev.laptop.org/git.do?p=olpc-2.6;a=history;f=drivers/video/olpc_dcon.h
- Getting started programming#Mode-switching_code_for_the_screen
- A video with closeups of an XO and ThinkPad screen, made using an XO microscope.